React-Hookz
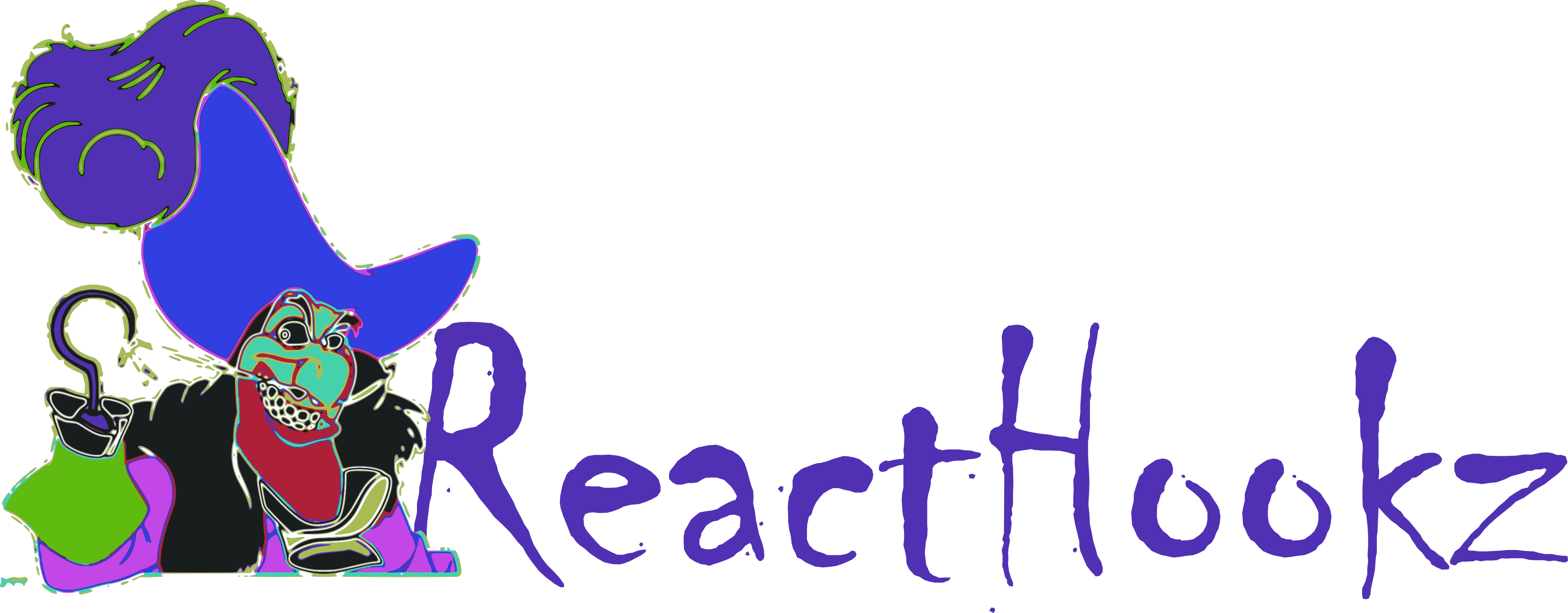
Installation
``` npm install react-hookz ```Usage
Minimal example:
Actions
```javascript export const addToCounter = (store: any, amount: number) => { const counter = store.state.counter + amount; store.setState({ counter }); }; ```HOC
```javascript import React from "react"; import ReactHookz from "react-hookz"; import as actions from "../actions/index"; export interface GlobalState { counter: number; } const initialState: GlobalState = { counter: 1 }; const useReactHookz = ReactHookz(React, initialState, actions); export const connect = Component => { return props => {let [state, actions] = useReactHookz();
let _props = { ...props, state, actions };
return <Component {..._props} />;
};
};
export default useReactHookz;
```
Component
```javascript import React from "react"; import { connect } from "../store"; interface Props { state: any; actions: any; } const Counter: React.FC = props => { const { state, actions } = props; return (<div className="Counter">
<p>
FC Counter:
{state.counter}
</p>
<button type="button" onClick={() => actions.addToCounter(1)}>
+1 to global
</button>
</div>
);
};
export default connect(Counter);
```